SuperScript
This documentation is still being written. Please bear with us while we complete this documentation.
Declarations
Along with emitters, declarations are a core concept of SuperScript.
What is a Declaration?
As described in Core Concepts, a declaration is an object which represents a server-side form of an entity to be used client-side.
A simple example might be a JavaScript variable representing a username: the name and value of the variable can be easily set server-side,
as well as whether to prepend var
.
Another example might be an HTML template which is modified server-side, then appended to the HTML to be used client-side.
Declarations are added to an application-wide Collection
.
Consequently, declarations may be added, retrieved, modified or deleted from anywhere in the application.
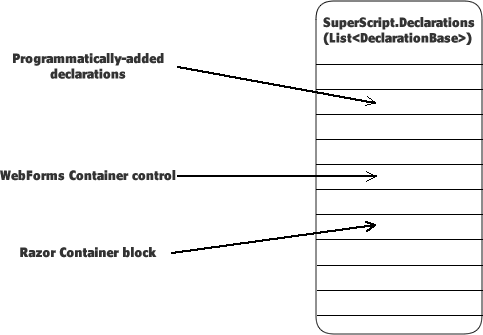
The figure above shows that wherever declarations are made, they are all added to the same collection, SuperScript.Declarations.Collection
.
Declaration Types
All declarations must be an instance of the abstract class, DeclarationBase
.
This class belongs to the SuperScript.Common library
SuperScript.Common contains no usable implementations of
DeclarationBase
. Rather, implementation-specific type can be found
in the various SuperScript add-on libraries.
DeclarationBase Members
A declaration is an instance of DeclarationBase
.
abstract class DeclarationBase { string EmitterKey { get; set; } string Name { get; set; } abstract string ToString(); }
Let's take a look at each of the members on the DeclarationBase
abstract class.
Name | Type | Description |
---|---|---|
EmitterKey | string |
Indicates which instance of
If not specified then the contents will be added to the default implementation of |
Name | string |
Gets or sets the name assigned to this declarable. |
ToString | string |
A call to this method will return this instance as a formatted string. |
Some of the libraries listed on the Current Projects page will contain implementations of
DeclarationBase
with additional, implementation-specific
properties.
Adding a Declaration
SuperScript.Declarations
offers two methods for adding implementations of
DeclarationBase
to
Collection
.
public static T AddDeclaration<T>(DeclarationBase declaration, int? insertAt = null) where T : DeclarationBase
Parameter | Type | Description |
---|---|---|
declaration | DeclarationBase |
A custom implementation of the |
insertAt | Nullable<int> |
Permits the instance of
The default value is |
public static IEnumerable<T> AddDeclarations<T>(IEnumerable<T> declarations) where T : DeclarationBase
Parameter | Type | Description |
---|---|---|
declarations | DeclarationBase |
A collection of custom implementations of the |
Removing a Declaration
SuperScript.Declarations
offers three methods for removing implementations of
DeclarationBase
from
Collection
.
public static void Remove(string nameOrCall)
public static void Remove(DeclarationBase declaration)
Parameter | Type | Description |
---|---|---|
declaration | DeclarationBase |
The instance of |
In addition to the two methods which remove a specific instance of DeclarationBase
,
it is also possible to reset the Collection
.
When resetting the Collection
, it is possible to
remove only the instances of DeclarationBase
which
were added in the code, leaving any configured instances of DeclarationBase
intact.
public static void Reset(bool removeDefaults = false)
Parameter | Type | Description |
---|---|---|
removeDefaults | bool |
Determines whether the configured default instances of
The default value is |
What Next?
Read about emitters here.